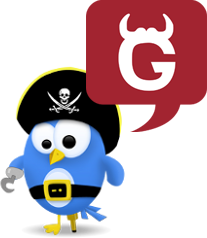
30/11/2016: Anontwi has been added to GNU (fsf.org).
01/09/2015: AnonTwi v1.1b released.
20/08/2015: Added support for GNUSocial.
14/05/2015: Added support for New API of Twitter (v1.1).
Anontwi - is a tool for OAuth2 applications (such as: GNUSocial, Twitter...) that provides different
layers of encryption and privacy methods.
![]() + Twitter PoC: ![]()
$ git clone https://github.com/epsylon/anontwi |
#!/usr/bin/python # -*- coding: iso-8859-15 -*- """ $Id$ Copyright (c) 2012/2015 psy 'epsylon@riseup.net' anontwi is free software; you can redistribute it and/or modify it under the terms of the GNU General Public License as published by the Free Software Foundation version 3 of the License. anontwi is distributed in the hope that it will be useful, but WITHOUT ANY WARRANTY; without even the implied warranty of MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU General Public License for more details. You should have received a copy of the GNU General Public License along with anontwi; if not, write to the Free Software Foundation, Inc., 51 Franklin St, Fifth Floor, Boston, MA 02110-1301 USA """ ################################################################### # See https://en.wikipedia.org/wiki/HMAC#Implementation # Example written by: michael@briarproject.org ################################################################### # Constants for AES256 and HMAC-SHA1 KEY_SIZE = 32 BLOCK_SIZE = 16 MAC_SIZE = 20 from os import urandom from hashlib import sha1, sha256 from Crypto.Cipher import AES from base64 import b64encode, b64decode trans_5C = "".join([chr (x ^ 0x5c) for x in xrange(256)]) trans_36 = "".join([chr (x ^ 0x36) for x in xrange(256)]) def hmac_sha1(key, msg): if len(key) > 20: key = sha1(key).digest() key += chr(0) * (20 - len(key)) o_key_pad = key.translate(trans_5C) i_key_pad = key.translate(trans_36) return sha1(o_key_pad + sha1(i_key_pad + msg).digest()).digest() def derive_keys(key): h = sha256() h.update(key) h.update('cipher') cipher_key = h.digest() h = sha256() h.update(key) h.update('mac') mac_key = h.digest() return (cipher_key, mac_key) def generate_key(): return b64encode(urandom(KEY_SIZE)) class Cipher(object): """ Cipher class """ def __init__(self, key="", text=""): """ Init """ self.block_size = 16 self.mac_size = 20 self.key = self.set_key(key) self.text = self.set_text(text) self.mode = AES.MODE_CFB def set_key(self, key): """ Set key """ # Base64 decode the key try: key = b64decode(key) except TypeError: raise ValueError # The key must be the expected length if len(key) != KEY_SIZE: raise ValueError self.key = key return self.key def set_text(self, text): """ Set text """ self.text = text return self.text def encrypt(self): """ Encrypt text """ # The IV, ciphertext and MAC can't be more than 105 bytes if BLOCK_SIZE + len(self.text) + MAC_SIZE > 105: self.text = self.text[:105 - BLOCK_SIZE - MAC_SIZE] # Derive the cipher and MAC keys (cipher_key, mac_key) = derive_keys(self.key) # Generate a random IV iv = urandom(BLOCK_SIZE) # Encrypt the plaintext aes = AES.new(cipher_key, self.mode, iv) ciphertext = aes.encrypt(self.text) # Calculate the MAC over the IV and the ciphertext mac = hmac_sha1(mac_key, iv + ciphertext) # Base64 encode the IV, ciphertext and MAC return b64encode(iv + ciphertext + mac) def decrypt(self): """ Decrypt text """ # Base64 decode try: iv_ciphertext_mac = b64decode(self.text) except TypeError: return None # Separate the IV, ciphertext and MAC iv = iv_ciphertext_mac[:BLOCK_SIZE] ciphertext = iv_ciphertext_mac[BLOCK_SIZE:-MAC_SIZE] mac = iv_ciphertext_mac[-MAC_SIZE:] # Derive the cipher and MAC keys (cipher_key, mac_key) = derive_keys(self.key) # Calculate the expected MAC expected_mac = hmac_sha1(mac_key, iv + ciphertext) # Check the MAC if mac != expected_mac: return None # Decrypt the ciphertext aes = AES.new(cipher_key, self.mode, iv) return aes.decrypt(ciphertext) if __name__ == "__main__": key = generate_key() print 'Key:', key # Encrypt and decrypt a short message text = 'Hello world!' c = Cipher(key, text) msg = c.encrypt() c.set_text(msg) print '\nCiphertext:', msg print 'Length:', len(msg) print 'Plaintext:', c.decrypt() # Encrypt and decrypt a long message text = 'Gosh this is a long message, far too long to fit in a tweet I dare say, especially when you consider the encryption overhead' c = Cipher(key, text) msg = c.encrypt() c.set_text(msg) print '\nCiphertext:', msg print 'Length:', len(msg) print 'Plaintext:', c.decrypt() # Check that modifying the message invalidates the MAC text = 'Hello world!' c = Cipher(key, text) msg = c.encrypt() msg = msg[:16] + msg[17] + msg[16] + msg[18:] c.set_text(msg) print '\nCiphertext:', msg print 'Length:', len(msg) print 'Plaintext:', c.decrypt()
AnonTwi runs on many platforms. It requires Python and the following libraries: - python-crypto - cryptographic algorithms and protocols for Python - python-httplib2 - comprehensive HTTP client library written for Python - python-pycurl - python bindings to libcurl - python-glade2 - GTK+ bindings: Glade support On Debian-based systems (ex: Ubuntu), run: - directly: sudo apt-get install python-crypto python-httplib2 python-pycurl python-glade2 - using setup-tools (http://pypi.python.org/pypi/setuptools): easy_install "packages" On Windows systems, is working (tested!) with: - python 2.7 - http://www.python.org/getit/ - pycrypto 2.3 - http://www.voidspace.org.uk/downloads/pycrypto-2.3.win32-py2.7.zip - httplib2 0.7.4 - http://httplib2.googlecode.com/files/httplib2-0.7.4.zip - pycurl 7.19.5.1 - http://pycurl.sourceforge.net/download/ - pygtk 2.24 - http://www.pygtk.org/downloads.html - using setup-tools (http://pypi.python.org/pypi/setuptools): easy_install.exe "packages"
------------------------ "Consumer" keys: ------------------------ + To use OAuth you need this tokens: 'consumer key' and 'consumer secret'.
- 1) Create a third party APP on your profile: + GNU/Social
: - Login to your account - Go to: Settings - Click on: "Register an OAuth client application" - Click on: "Register a new application" */settings/oauthapps/new - Fill form correctly * Icon: You can use AnonTwi website logo * Name: (ex: AnonTwi -IMPORTANT!!) -> You must enter a unique name, no entered by others before. Try for example random strings. Ex: AnonTwi27523561361) * Description: (ex: Anontwi -GNU/Social edition-) * Source URL: (ex: http://anontwi.03c8.net) * Organization: (ex: AnonTwi) * Homepage: (ex: http://anontwi.03c8.net) * Callback URL: (ex: http://anontwi.03c8.net) * Type of Application: Desktop * Default access for this application: Read-Write + Twitter
: - Login to your account - Go to: https://apps.twitter.com/ - Click on: "Create New App" * https://apps.twitter.com/app/new- Fill form correctly * Name: (ex: AnonTwi -IMPORTANT!!) -> You must enter a unique name, no entered by others before. Try for example random strings. Ex: AnonTwi27523561361) * Description: (ex: Anontwi -GNU/Social edition-) * Website: (ex: http://anontwi.03c8.net) * Callback URL: (ex: http://anontwi.03c8.net)
- 2) Get your OAuth settings: Click on the name of your new APP connector (ex: AnoNTwi) + GNU/Social:![]()
+ Twitter:
![]()
![]()
![]()
- Open "config.py" with a text editor, and enter tokens (below!)- Remember: * If you go to use shell mode, you should generate your tokens with command: --tokens * For connect using TOR add: --proxy "http://127.0.0.1:8118" - Run ./anontwi or python anontwi (To use interface: ./anontwi --gtk) --------------------- "Token" keys: --------------------- + To use OAuth you need this tokens: 'token key' and 'token secret'. - Launch: ./anontwi --tokens - Follow the link to read your "PinCode" + GNU/Social:
![]()
+ Twitter:
![]()
- Enter your PinCode - After a few seconds, you will reviece a response like this: "Generating and signing request for an access token Your Twitter Access Token key: xxxxxxxx-xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx Access Token secret: xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx" + With these tokens, you can start to launch -AnonTwi- commands like this: ./anontwi [-m 'text' | -r 'ID' | -d @user | -f @nick | -u @nick] [OPTIONS] 'token key' 'token secret' + Remember that you can EXPORT tokens like environment variables to your system, to don't use them every time If you did it, you can start to launch -AnonTwi- commands like this: ./anontwi [-m 'text' | -r 'ID' | -d @user | -f @nick | -u @nick] [OPTIONS]
+ To remember: - Connections to API are using fake headers automatically - To launch TOR, add this command: --proxy "http://127.0.0.1:8118" - Check if you are doing geolocation in your messages (usually is 'off' by default) - You can generate 'token key' and 'token secret' every time that you need - View output results with colours using parameter: --rgb (better with obscure backgrounds) - Use --gen to generate STRONG PIN/keys (ex: --pin '1Geh0RBm9Cfj82NNhuQyIFFHR8F7fI4q7+7d0a3FrAI=') - Try to add encryption to your life :) ----------------------------------- Retrieve you API tokens, using TOR: ----------------------------------- ./anontwi --tokens --proxy "http://127.0.0.1:8118" ---------------------------------------------------- Generate PIN key for encrypting/decrypting messages: ---------------------------------------------------- ./anontwi --gen PIN key: K7DccSf3QPVxvbux85Tx/VIMkkDkcK+tFzi45YZ5E+g= Share this key privately with the recipients of your encrypted messages. Don't send this key over insecure channels such as email, SMS, IM or Twitter. Use the sneakernet! ;) ---------------------- Launch GTK+ Interface: ---------------------- Enjoy visual mode experience ;) ./anontwi --gtk ------------------------ Launch an IRC bot slave: ------------------------ Launch it and you will have a bot slave waiting your orders on IRC. ./anontwi --irc='nickname@server:port#channel' If you don't put a nickname or a channel, AnonTwi will generate randoms for you :) ./anontwi --irc='irc.freenode.net:6667' ------------------------ Short an url, using TOR: ------------------------ ./anontwi --short "url" --proxy "http://127.0.0.1:8118" ----------------------------------- Send a plain-text tweet, using TOR: ----------------------------------- ./anontwi -m "Hello World" --proxy "http://127.0.0.1:8118" ------------------------ Send an encrypted tweet: ------------------------ ./anontwi -m "Hello World" --enc --pin "K7DccSf3QPVxvbux85Tx/VIMkkDkcK+tFzi45YZ5E+g=" -------------- Remove a tweet: --------------- You need the ID of the tweet that you want to remove. - launch "--tu @your_nick 'num'" to see tweets IDs of your timeline. ./anontwi --rm-m "ID" ------------------ Retweet a message: ------------------ You need the ID of the tweet that you want to RT. - launch "--tu @nick 'num'" to see tweets IDs of a user. ./anontwi -r "ID" ------------------------------------------------- Send a plain-text DM (Direct Message), using TOR: ------------------------------------------------- ./anontwi -m "See you later" -d "@nick" --proxy "http://127.0.0.1:8118" --------------------- Send an encrypted DM: --------------------- ./anontwi -m "See you later" -d "@nick" --enc --pin "K7DccSf3QPVxvbux85Tx/VIMkkDkcK+tFzi45YZ5E+g=" --------------- Remove a DM: --------------- You need the ID of the DM that you want to remove. - launch "--td 'num'" to see Direct Messages IDs of your account. ./anontwi --rm-d "ID" -------------------------------------- Send a media message, using TOR: -------------------------------------- Twitter will show your media links. For example, if you put a link to an image ./anontwi -m "https://host/path/file.jpg" --proxy "http://127.0.0.1:8118" ---------------------------------------- Send reply in a conversation, using TOR: ---------------------------------------- You need the ID of the message of the conversation. - launch "--tu @nick 'num'" to see tweets IDs of a user timeline. - launch "--tf 'num'" to see tweets IDs of your 'home'. Add names of users that participates on conversation at start of your message. ./anontwi -m "@user1 @user2 text" --reply "ID" --proxy "http://127.0.0.1:8118" --------------------------------- Send a friend request, using TOR: --------------------------------- ./anontwi -f "@nick" --proxy "http://127.0.0.1:8118" ---------------------------------- Stop to follow a user, using TOR: ---------------------------------- ./anontwi -u "@nick" --proxy "http://127.0.0.1:8118" ---------------------------------- Create a favorite, using TOR: ---------------------------------- ./anontwi --fav "ID" --proxy "http://127.0.0.1:8118" ---------------------------------- Destroy favorite, using TOR: ---------------------------------- ./anontwi --unfav "ID" --proxy "http://127.0.0.1:8118" ------------------------ Block a user, using TOR: ------------------------ ./anontwi --block "@nick" --proxy "http://127.0.0.1:8118" -------------------------- Unblock a user, using TOR: -------------------------- ./anontwi --unblock "@nick" --proxy "http://127.0.0.1:8118" ----------------------------------------- Show a number of recent tweets of a user: ----------------------------------------- You can control number of tweets to be reported. For example, 10 most recent tweets is like this: ./anontwi --tu "@nick 10" ------------------------------------------------------- Show a number of recent tweets of your 'home' timeline: ------------------------------------------------------- You can control number of tweets to be reported. For example, 10 most recent tweets is like this: ./anontwi --tf "10" ------------------------------------------------------- Show a number of recent favorites ------------------------------------------------------- You can control number of tweets to be reported. For example, 10 most recent tweets is like this: ./anontwi --tfav "@nick 10" ---------------------------------- Split a long message into "waves": ---------------------------------- Very usefull if you want to send long messages. It uses Twitter restrictions as much efficient as possible. Encryption is allowed :) ./anontwi -m "this is a very long message with more than 140 characters..." --waves ---------------------------------- Send fake geolocation coordenates: ---------------------------------- If you dont put any (--gps), coordenates will be random :) ./anontwi -m "text" --gps "(-43.5209),146.6015" ------------------------------------------------- Show a number of Direct Messages of your account: ------------------------------------------------- You can control number of DMs to be reported. For example, 5 most recent DMs is like this: ./anontwi --td "5" ------------------------------------------- Returns global Trending Topics, using TOR: ------------------------------------------- ./anontwi --tt --proxy "http://127.0.0.1:8118" ------------------------------------------- Returns last mentions about you, using TOR: ------------------------------------------- You can control number of tweets to be reported. For example last recent tweet: ./anontwi --me "1" --proxy "http://127.0.0.1:8118" --------------------------------------------- Decrypt a tweet directly from URL, using TOR: --------------------------------------------- Remeber, to decrypt, you need the PIN/Key that another user has used to encrypt the message (symmetric keys) To decrypt you don't need 'token key' and 'token secret' :) ./anontwi --dec "http://twitter.com/encrypted_message_path" --pin "K7DccSf3QPVxvbux85Tx/VIMkkDkcK+tFzi45YZ5E+g=" --proxy "http://127.0.0.1:8118" ---------------------------------------- Decrypt a tweet directly from raw input: ---------------------------------------- Remeber, to decrypt, you need the PIN/Key that another user has used to encrypt the message (symmetric keys) To decrypt you don't need 'token key' and 'token secret' :) ./anontwi --dec "7asNGpFFDKQl7ku9om9CQfEKDq1ablUW+srgaFiEMa+YK0no8pXsx8pR" --pin "K7DccSf3QPVxvbux85Tx/VIMkkDkcK+tFzi45YZ5E+g=" ---------------------------------------------------------- Save tweets starting from the last (max: 3200), using Tor: ---------------------------------------------------------- You can control number of tweets to be reported. For example last 1000 tweets: ./anontwi --save "1000" --proxy "http://127.0.0.1:8118" ------------------------------------------------- Save favorites starting from the last, using Tor: ------------------------------------------------- You can control number of tweets to be reported. For example last 100 tweets: ./anontwi --sfav "@nick 100" --proxy "http://127.0.0.1:8118" ------------------- Suicide, using TOR: ------------------- This will try to delete your tweets, your DMs and if is possible, your account. ./anontwi --suicide --proxy "http://127.0.0.1:8118"
AnonTwi is released under the terms of the General Public License v3 and is copyrighted by psy.
psy - GPG Public ID Key: 0xB8AC3776
If you want to contribute to AnonTwi development, reporting a bug,
providing a patch, commenting on the code base or simply need to find help
to run AnonTwi, first refer to:
irc.freenode.net - #AnonTwi
If nobody gets back to you, then drop me an e-mail.
This -framework- is actively looking for new sponsors and funding.
If you or your organization has an interest in keeping AnonTwi, please contact directly.
To make donations use the following hashes:
- Bitcoin: 19aXfJtoYJUoXEZtjNwsah2JKN9CK5Pcjw
- Ecoin: 6enjPY7PZVq9gwXeVCxgJB8frsf4YFNzVp